Loops can execute a block of code a number of times.
Loop allows students to connect with course content, their teachers and fellow learners. It's where students access their notes, participate in discussion fora, construct their ePortfolio and participate in webinars. Loop is an amalgamation of several different learning technologies into one platform. By creating this 'brand' loop, we take. LOOP LLC is a company whose primary business is offloading foreign crude oil from tankers, and storing & distributing offshore domestic crude oil.
JavaScript Loops
Loops are handy, if you want to run the same code over and over again, each time with a different value.
Often this is the case when working with arrays:
Instead of writing:
';
text += cars[1] + '
';
text += cars[2] + '
';
text += cars[3] + '
';
text += cars[4] + '
';
text += cars[5] + '
';
You can write:
for (i = 0; i < cars.length; i++) {
text += cars[i] + '
';
}
Different Kinds of Loops
JavaScript supports different kinds of loops:
for
- loops through a block of code a number of timesfor/in
- loops through the properties of an objectfor/of
- loops through the values of an iterable objectwhile
- loops through a block of code while a specified condition is truedo/while
- also loops through a block of code while a specified condition is true
The For Loop
The for
loop has the following syntax:
// code block to be executed
}
Statement 1 is executed (one time) before the execution of the code block.
Statement 2 defines the condition for executing the code block.
Statement 3 is executed (every time) after the code block has been executed.
Example
text += 'The number is ' + i + '
';
}
From the example above, you can read:
Statement 1 sets a variable before the loop starts (var i = 0).
Statement 2 defines the condition for the loop to run (i must be less than 5).
Statement 3 increases a value (i++) each time the code block in the loop has been executed.
Statement 1
Normally you will use statement 1 to initialize the variable used in the loop (i = 0).
This is not always the case, JavaScript doesn't care. Statement 1 is optional.
You can initiate many values in statement 1 (separated by comma):
Example
text += cars[i] + '
';
}
And you can omit statement 1 (like when your values are set before the loop starts):
Example
var len = cars.length;
var text = ';
for (; i < len; i++) {
text += cars[i] + '
';
}
Statement 2
Often statement 2 is used to evaluate the condition of the initial variable.
This is not always the case, JavaScript doesn't care. Statement 2 is also optional.
If statement 2 returns true, the loop will start over again, if it returns false, the loop will end.
If you omit statement 2, you must provide a break inside the loop. Otherwise the loop will never end. This will crash your browser. Read about breaks in a later chapter of this tutorial.
Statement 3
Often statement 3 increments the value of the initial variable.
This is not always the case, JavaScript doesn't care, and statement 3 is optional.
Statement 3 can do anything like negative increment (i--), positive increment (i = i + 15), or anything else.
Statement 3 can also be omitted (like when you increment your values inside the loop):
Example
var len = cars.length;
for (; i < len; ) {
text += cars[i] + '
';
i++;
}
The For/In Loop
The JavaScript for/in
statement loops through the properties of an object:
Example
var text = ';
var x;
for (x in person) {
text += person[x];
}
The For/Of Loop
The JavaScript for/of
statement loops through the values of an iterable objects.
for/of
lets you loop over data structures that are iterable such as Arrays, Strings, Maps, NodeLists, and more.
The for/of
loop has the following syntax:
// code block to be executed
}
variable - For every iteration the value of the next property is assigned to the variable. Variable can be declared with const
, let
, or var
.
iterable - An object that has iterable properties.
Looping over an Array
Example
var x;
for (x of cars) {
document.write(x + '
');
}
Looping over a String
Example
Loopnet
var x;
for (x of txt) {
document.write(x + '
');
}
The While Loop
The while
loop and the do/while
loop will be explained in the next chapter.
Look up loop in Wiktionary, the free dictionary. |
Loop or LOOP may refer to:
Brands and enterprises[edit]
- Loop (mobile), a mobile virtual network operator in Bulgaria
- Loop, clothing a company founded by Carlos Vasquez in the 1990s and worn by Digable Planets
- Loop Mobile, a mobile phone operator in India
- Loop (shopping service), a reusable container program announced in 2019 by TerraCycle
Geography[edit]
- Loop (Texarkana), a roadway loop around Texarkana, Arkansas
- Loop, Germany, a municipality in Schleswig-Holstein, Germany
- Loop 101, see Arizona State Route 101, a semi-beltway of the Phoenix Metropolitan Area
- Loop 202, see Arizona State Route 202, a semi-beltway of the Phoenix Metropolitan Area
- Loop 303, see Arizona State Route 303, a semi-beltway of the Phoenix Metropolitan Area
- Chicago Loop, the downtown neighborhood of Chicago bounded by the elevated railway The Loop (CTA)
- Loop Retail Historic District, a shopping district in the Chicago Loop
- Delmar Loop, an entertainment district in St. Louis, Missouri
- London Outer Orbital Path (or LOOP), a signed walk around the edge of Outer London, England
- Louisiana Offshore Oil Port (or LOOP), a deep-water port in the Gulf of Mexico off the coast of Louisiana
People[edit]
- Call Me Loop (born 1991), English singer and songwriter
- Liza Loop, American technology pioneer
- Uno Loop (born 1930), Estonian singer, musician, athlete, actor, and educator
Arts, entertainment, and media[edit]
Film[edit]
- Loop (1997 film), a British romantic comedy
- Loop (1999 film), a Venezuelan film
- Loop (2020 film), an American animated short
- Film loop, the slack portion of the film around the projector lens in a movie projector
- Porn loop, an 8 or 16 mm video 'short' of a pornographic nature that could be purchased from men's magazines starting in the 1950s
Music[edit]
Groups[edit]
- Loop (band), a London rock band
Other uses in music[edit]
- Loop (album), a 2002 album by Keller Williams
- Loop (music), a finite element of sound which is repeated by technical means
- 'Loop' (song), a song by Maaya Sakamoto
Other uses in arts, entertainment, and media[edit]
- Loop (novel), a novel in the Ring series by Koji Suzuki
- LOOP Barcelona, an annual meeting point for video art in Barcelona, Spain
- Loop Mania, a mobile arcade video game
Computing and technology[edit]
- Loop (computing), a method of control flow in computer science
- LOOP (programming language), the pedagogical primitive recursive programming language with bounded loops
- Loop (telecommunication), sending a signal on a channel and receiving it back at the sending terminal
- Audio induction loop, an aid for the hard of hearing
- Local loop, (aka local tail, subscriber line, or the last mile), the physical link in telephony that links the customer premises to the telephone_company (telco)
- Loop device, a Unix device node that allows a file to be mounted as if it were a device
- LOOPS, the object system for Interlisp-D
Mathematics[edit]
Loop Yarn
- Loop (algebra), a quasigroup with an identity element
- Loop (graph theory), an edge that begins and ends on the same vertex
- Loop (topology), a single point of a path that starts and ends at the same point
Sports[edit]
- Loop, a type of playboating maneuver
- Aerobatic loop, a type of aircraft aerobatic maneuver
- Flight (cricket) or loop, an aspect of bowling in cricket
- Loop jump, a figure skating jump
- Loop, a type of offensive shot in table tennis
Roller coasters[edit]
- Loop (roller coaster), a basic roller coaster inversion
- Pretzel loop, a roller coaster element
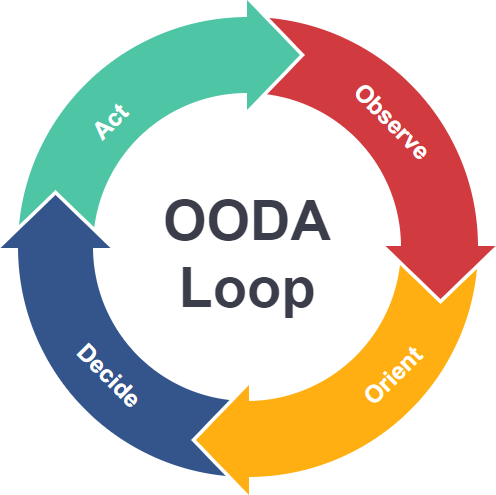
Transportation[edit]
- Loop (Amtrak train), a discontinued Amtrak train
- The Loop (CTA), a rapid transit section bounding Chicago's Loop neighborhood
- Loop, a DC-to-Baltimore underground public transport system proposed by The Boring Company
- Balloon loop, a section of track that allows reversal of direction without stopping
- Loop line (railway), a branch line that deviates from a direct route and rejoins it at another location
- Loop route, a main route or highway that forms a closed loop
- Passing loop, a short section of track that allows trains to pass on a single track route
- Ring road or loop, a main route or highway that encircles a town or city
- Spiral (railway), a section of track that allows a train to climb a steep hill
Loops And Threads Yarn
Other uses[edit]
- Loop (biochemistry), a flexible region in a protein's secondary structure
- Loop (education), the process of advancing an elementary school teacher with his or her class
- Loop (knot), one of the fundamental structures used to tie knots
- Loop, a cul de sac
- Loop, a type of fingerprint pattern
See also[edit]
- Loupe, a small magnifying glass used by jewelers, watchmakers, and other precision craftsmen
- Line echo wave pattern (LEWP)
Looperman.com

Transportation[edit]
- Loop (Amtrak train), a discontinued Amtrak train
- The Loop (CTA), a rapid transit section bounding Chicago's Loop neighborhood
- Loop, a DC-to-Baltimore underground public transport system proposed by The Boring Company
- Balloon loop, a section of track that allows reversal of direction without stopping
- Loop line (railway), a branch line that deviates from a direct route and rejoins it at another location
- Loop route, a main route or highway that forms a closed loop
- Passing loop, a short section of track that allows trains to pass on a single track route
- Ring road or loop, a main route or highway that encircles a town or city
- Spiral (railway), a section of track that allows a train to climb a steep hill
Loops And Threads Yarn
Other uses[edit]
- Loop (biochemistry), a flexible region in a protein's secondary structure
- Loop (education), the process of advancing an elementary school teacher with his or her class
- Loop (knot), one of the fundamental structures used to tie knots
- Loop, a cul de sac
- Loop, a type of fingerprint pattern
See also[edit]
- Loupe, a small magnifying glass used by jewelers, watchmakers, and other precision craftsmen
- Line echo wave pattern (LEWP)